Fine-grained authorization for your Node.js apps
Access controls in Node.js
Authorization in Node.js
Node.js is a popular, open-source Javascript server runtime environment. Aserto is a powerful authorization-as-a-service platform that lets you add resource-level, real-time authorization to your Node.js applications in under a day. Quickstarts, a Node / Express.js SDK, and REST/gRPC APIs are available to help you get up and running.
Aserto helps you define, evolve, and enforce user permissions within your Node.js applications. It’s simple to integrate, but powerful enough to support fine-grained access control, granular permissions, and audit trails.
Built on trusted open-source projects like OPA and OCI, Aserto handles all the heavy lifting required to achieve secure, scalable, high-performance multi-tenant RBAC, attribute-based access control (ABAC), and relationship-based access controls (ReBAC).
Adding authorization to your Node.js application
Authorization should happen every time your application needs to access a protected resource on behalf of a user. To perform an authorization check, your application should call the Aserto Authorizer and pass user context (typically in the form of an access token), policy context, and resource context. The authorizer uses the popular open-source OPA decision engine to quickly make an authorization decision based on this data. The decision returned should determine whether your application should pass or fail the request.
The Aserto Node.js SDK contains three capabilities that make it easy to add authorization to your application:
- A function called
is
which you can call with the user context, policy context, and resource context, and that returns an authorization decision. - Middleware that can be added to an Express.js route, which calls the Aserto authorizer and will return an “access denied” response if the user isn’t authorized to perform the operation on that resource.
- A display state map for every operation, which your frontend (e.g. React) application can use to conditionally render UI based on the permissions of the current user.
Authorizer deployment options
Aserto runs a hosted authorizer as a service, which is great for development, since you don’t have to stand anything up to get started with adding authorization to your application.
For production deployments, you should deploy the authorizer right next to your application, either as a sidecar or a microservice in the same cluster or subnet. Aserto’s control plane automatically syncs all of the user, resource, and policy data to connected authorizers in near real-time, so it is has the most up-to-date data to make lightning-fast authorization decisions for your Node.js application.
Auditing authorization decisions
Aserto automatically captures a record of every authorization decision in a decision log. These logs can be streamed or batched up into your favorite log analysis tool or SIEM. Aserto makes this data available to applications in two ways:
1. A set of bucket storage objects you can download
2. A near real-time data stream you can tap via the Aserto APIs or the Aserto CLI
Open-source authorizer
Authorization is on the critical path of every application request, so response time is critical. The Aserto open-source authorizer is known as Topaz. The authorizer is deployed as microservices or sidecars right next to your application to minimize latency and maximize availability, so your application is never dependent on the availability of a remote authorization service.
The Topaz authorizer make decisions based on three important inputs:
1. Policies from your policy registry
2. Users, attributes, and roles from Identity provider, supplemented with application-specific information from the Aserto directory
3. Resource context passed by your application
Any changes made to users, attributes, policy, or resources and synced with the Aserto directory, so your authorization decisions are never based on stale data. Aserto pushes these updates to your locally deployed authorizers in near real time. Alternatively, the system can be configured to update on an interval determined by the application administrator.
Leveraging the policy, user, and resource contexts, makes it easy to evolve your authorization from coarse-grained RBAC to fine-grained authorization using ABAC, relationship-based access controls (ReBAC), or combinations to meet your evolving requirements. You can build a strong authorization foundation once, and adapt your access control model over time to match your customers’ requirements.
Aserto also comes with the following benefits:
- Out-of-the-box support for audit trails, custom roles, RBAC, ABAC, and relationship-based access controls (ReBAC)
- Open-source authorizer you can deploy in your cloud today
- A policy-as-code workflow to build, tag, push, version, and pull policy images (like docker images)
- Integrations with your identity provider, artifact registry, logging system, and frontend framework of your choice.
- Built on top of a trusted OSS policy engine, the Open Policy Agent (OPA)
Resources
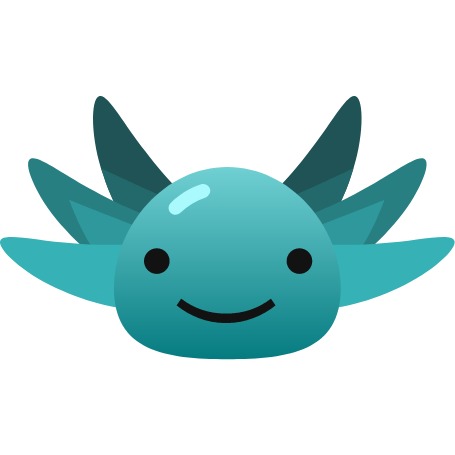