Fine-grained authorization service for Go apps
Access controls in Golang
Authorization in Golang
Golang, sometimes referred to as Go, is an open-source, compiled, and statically typed programming language created by Google. Aserto is a fully customizable application authorization service that brings fine-grade access controls to your Golang application.
Aserto helps you define, evolve, and enforce user permissions within Golang apps, making authorization as easy as an API call. Aserto’s quickstarts, Golang SDK, and REST, gRPC, and GraphQL APIs make it simple to integrate and easy to get up and running. Integration typically takes less than a day, from which point it delivers fine-grained, resource-level access control and audit trails - all of which are necessary features for serving enterprise customers.
Built on trusted open-source projects like OPA, OCI, and Sigstore, Aserto brings secure, scalable, high-performance role-based access control (RBAC), attribute-based access control (ABAC), and relationship-based access controls (ReBAC) to Golang applications.
Why use Aserto over libraries like Casbin or GoRBAC?
There are a few key differences that sets Aserto apart from the Go libraries you may be familiar with.
Authorize locally, manage centrally
Libraries like Casbin or GoRBAC are embedded directly into the application. While authorization is a local operation, each microservice has to define its own authorization policy and manage all of the data that is used for authorization. It also needs to capture and handle decision logs, and aggregate them somewhere.
The Aserto authorization service handles all of this for you: it allows you to manage authorization policies, user data, and resource data in one central place, and automatically captures and aggregates decision logs. At the same time, Aserto authorizers are deployed right next to your application, so authorization decisions happen at the same low-latency and high-availability as if you were using a library.
If your application consists of multiple services and runs multiple instances of these services, this benefit becomes even more crucial: central management of all the authorization artifacts and decision logs is the only way to scale the operation of a modern microservices architecture.
Separation of concerns
In Aserto, authorization policy is extracted out of the application logic, and stored/versioned as its own code artifact. This separation of concerns allows security engineers to evolve the authorization policy separately from the application code.
Authorization policies are authored in Rego, an open source policy language that allows declarative and flexible policy definitions. This means that developers can write concise policies and evolve them by changing the rules in the policy, change-tracked using a source control system, rather than flipping rows in a database.
Lastly, Aserto is an externalized authorization service. This means that changes to policy take effect immediately and without the need to redeploy the app.
Users as first-class citizens
Users and roles are first-class citizens in Aserto. Our directory of users and their roles is continuously synchronized with the Aserto authorizer. This means Aserto can reason about users and roles as a part of the policy itself without requiring role resolution as an additional external step.
Having role resolution as part of the application introduces its own set of risks, including coding errors that may bring the wrong data to the decision engine. Having user and role information come from the Aserto directory eliminates that risk.
Setting things up
To get started you’ll want to leverage the aserto-go
package to add authorization middleware. This package has 2 components:
- An authorizer client, which is the low-level interface in communication with our authorization API. This is typically what you’d use to create the middleware.
- HTTP and/or gRPC middleware, which focuses the responsibility of making authorization decisions to a single component rather than fragmenting the logic across multiple routes or service methods.
Deployment options
The Authorizer is an authorization engine built on top of the open source Open Policy Agent (OPA) decision engine. Your Go app can interact with the Authorizer via the aserto-go
package via gRPC or HTTPS REST APIs. Creating a tenant in Aserto will automatically create a corresponding hosted Authorizer instance which you can use to develop and test.
For your production workloads, the authorizer can be deployed as a sidecar, or local service right next to your application to provide minimal latency and 100% availability tied to your application’s uptime.
Decision logging
Out-of-box decision logging creates an easy audit trail for security and compliance. All decisions made by the Aserto authorizer can be easily viewed and tracked via the Aserto control plane.
Topaz authorizer
The authorizer is known as Topaz. It is an open-source authorization project that supports every authorization model out of the box.
Topaz makes authorization decisions based on three important inputs:The authorizer makes authorization decisions based on three important inputs:
1. Policies from your policy registry
2. Users, attributes, and roles from your identity provider (e.g. Okta, Auth0, etc.) supplemented with application-specific information stored in the Aserto directory
3. Resource context passed by in your application
Any changes made to users, attributes, policy, or resources are automatically synchronized into the Aserto directory. The control plane pushes updates to local authorizers in near real-time, ensuring your decisions are based on up-to-date information.
Aserto comes with the following benefits:
- Out-of-the-box support for audit trails, custom roles, RBAC, ABAC, and ReBAC
- Open-source authorizer that you can deploy in your cloud today
- Use a policy-as-code workflow to build, tag, push, version, and pull policy images just like docker images
- Quick onboarding via first-class citizen integrations with your identity provider, artifact registry, logging system, backend programming language, and frontend framework.
- Built on top of a trusted open source policy engine, the Open Policy Agent (OPA)
Resources
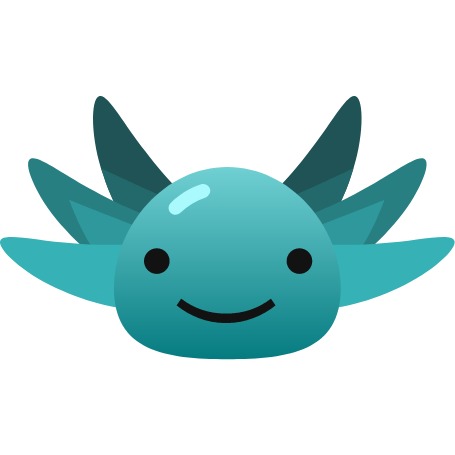